Salesforce Field Usage Analyzer
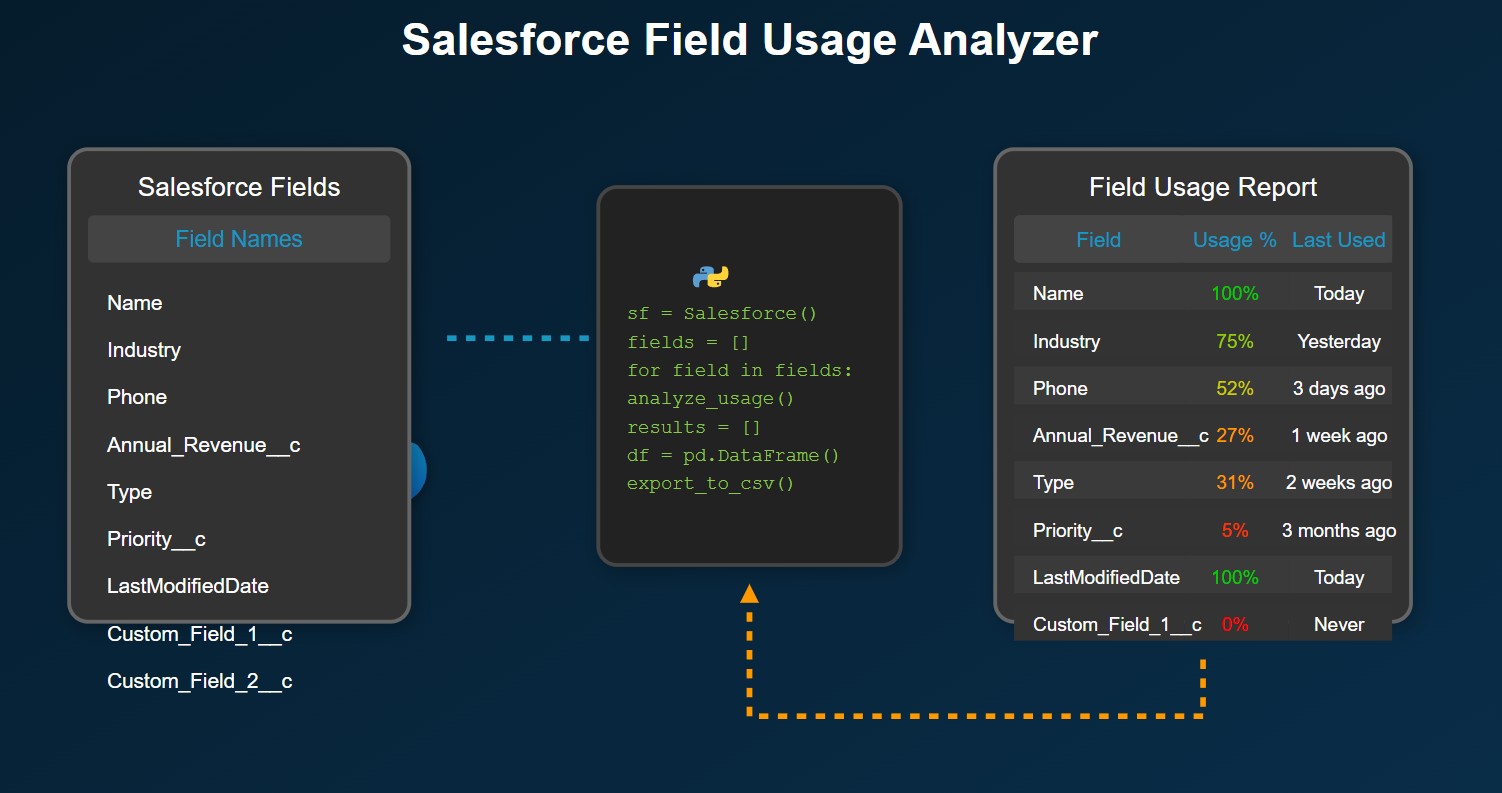
A Python tool that analyzes Salesforce object field usage statistics
The Problem
One of the biggest challenges when working with Salesforce is understanding how fields are actually being used in your org. As orgs grow, they often accumulate hundreds of custom fields—many of which may be rarely or never used.
This creates several issues:
- Cluttered page layouts that reduce user efficiency
- Confusing data models that make training difficult
- Excessive maintenance when making system changes
- Unnecessary storage costs for unused data
Salesforce doesn't provide built-in tools for easily analyzing field usage across records, making it difficult to know which fields can be safely removed or deprioritized.
The Solution
I built a Python application that:
-
Connects to Salesforce using the
simple-salesforce
library - Analyzes any Salesforce object to determine field usage
- Generates a CSV/Excel report showing the date of the last record using each field
- Calculates the percentage of records utilizing each field
- Helps administrators make data-driven decisions about field optimization
This tool has helped identify numerous unused fields in production orgs, allowing for cleaner layouts and more maintainable systems.
Technical Implementation
The application works by:
- Authenticating with Salesforce using admin credentials
- Retrieving metadata about the object's fields
- Constructing dynamic SOQL queries to analyze field usage
- Processing the results with Pandas for data manipulation
- Exporting a clean report for administrators to use in decision making
Sample Code
from simple_salesforce import Salesforce
import pandas as pd
import datetime
# Connect to Salesforce
sf = Salesforce(
password='your_password',
username='your_username',
organizationId='your_org_id'
)
# The object to analyze
object_name = 'Account'
# Get a list of all fields for the object
object_desc = getattr(sf, object_name).describe()
fields = [field['name'] for field in object_desc['fields']]
# Generate results
results = []
for field in fields:
# Query to find the most recent record using this field
query = f"SELECT Id, LastModifiedDate FROM {object_name} WHERE {field} != null ORDER BY LastModifiedDate DESC LIMIT 1"
records = sf.query(query)
# Count total records using this field
count_query = f"SELECT COUNT(Id) total FROM {object_name} WHERE {field} != null"
count_result = sf.query(count_query)
# Count all records
total_query = f"SELECT COUNT(Id) total FROM {object_name}"
total_result = sf.query(total_query)
# Calculate usage percentage
usage_percent = 0
if total_result['records'][0]['total'] > 0:
usage_percent = (count_result['records'][0]['total'] / total_result['records'][0]['total']) * 100
# Add to results
last_used_date = None
if records['totalSize'] > 0:
last_used_date = records['records'][0]['LastModifiedDate']
results.append({
'Field': field,
'Last Used': last_used_date,
'Usage Percentage': usage_percent
})
# Convert to DataFrame and export
df = pd.DataFrame(results)
df.to_csv(f'{object_name}_field_usage.csv', index=False)
View on GitHub
Check out the full code repository on GitHub to see implementation details, usage instructions, and to download the tool for your own Salesforce org.
GitHub RepositoryBusiness Impact
This tool has helped organizations:
- Reduce page layout complexity by up to 30%
- Streamline data maintenance processes
- Improve user adoption through cleaner interfaces
- Make data-driven decisions about org customizations
- Optimize storage usage in Salesforce
By providing clear insights into field usage patterns, administrators can confidently remove unnecessary fields and focus on those that provide real business value.